Input使うとキーボードやPadの複数対応が面倒になる。
Input Systemを使うとその辺を一括でまとめて扱えます。
Unity公式の説明はこちら:https://docs.unity3d.com/Packages/com.unity.inputsystem@1.7/manual/index.html
Unity 2022.3.22f1時点だとPackage Managerからインストールする必要があります。
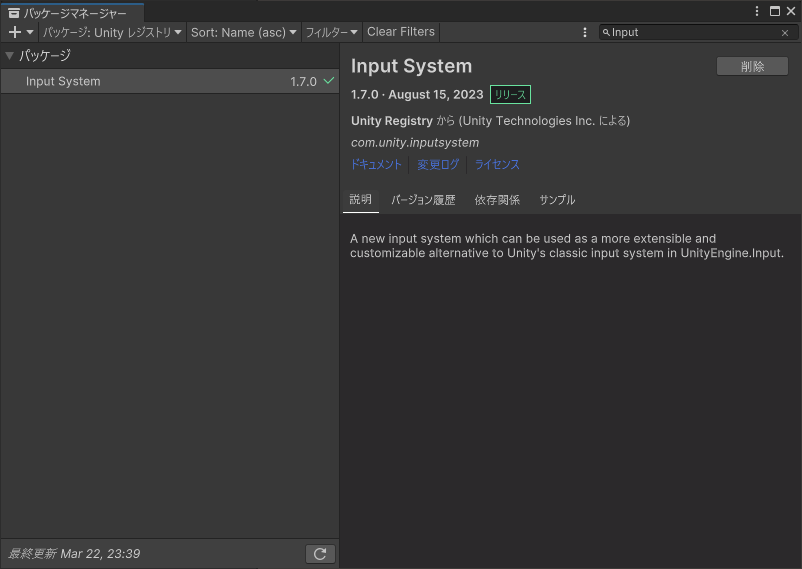
右クリック→作成→Input Actionsで作成。
作成したInput Actionsを開いて設定する。
※ 2D VectorはActionの「Control Type」を変更しないと選択肢に出てこないので注意。個人的によく忘れるポイント。
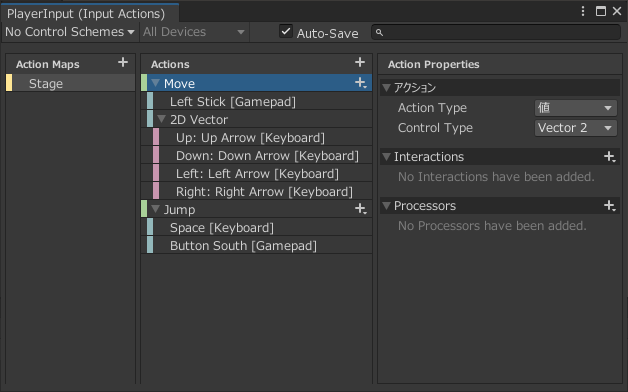
インスペクターの「Generate C# Class」を有効にして保存すると、Input ActionsがC#のソースに化けます。
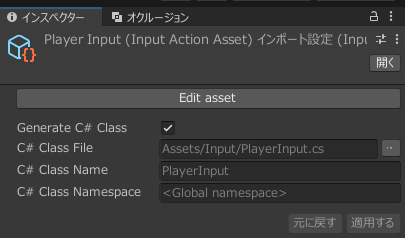
後はC#から利用するだけになります。
以下のコードは入力に応じてデバッグ出力するだけのコード。
using System.Collections;
using System.Collections.Generic;
using Unity.VisualScripting;
using UnityEngine;
using UnityEngine.InputSystem;
public class PlayerController : MonoBehaviour
{
private PlayerInput input;
void Start()
{
input = new PlayerInput();
input.Enable();
input.Stage.Move.performed += OnMovePerformed;
input.Stage.Move.canceled += OnMoveCancele;
input.Stage.Jump.performed += OnJump;
}
private void OnDisable(){
input.Disable();
input.Stage.Move.performed -= OnMovePerformed;
input.Stage.Move.canceled -= OnMoveCancele;
input.Stage.Jump.performed -= OnJump;
}
private void OnMovePerformed(InputAction.CallbackContext context){
Vector2 value = context.ReadValue<Vector2>();
Debug.Log( "Move : " + value );
}
private void OnMoveCancele(InputAction.CallbackContext context){
Debug.Log( "Stop" );
}
private void OnJump(InputAction.CallbackContext context){
Debug.Log( "Jump" );
}
}
コンソールでInput Systemの動作を確認できました。
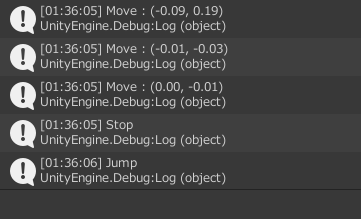